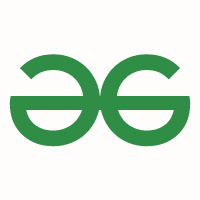
Write a program to reverse an array or string - GeeksforGeeks
Write a program to reverse an array or string
- Difficulty Level : Basic
- Last Updated : 08 Sep, 2020
Given an array (or string), the task is to reverse the array/string.
Examples :
Input : arr[] = {1, 2, 3}Output : arr[] = {3, 2, 1}Input : arr[] = {4, 5, 1, 2}Output : arr[] = {2, 1, 5, 4}
Recommended: Please solve it on “PRACTICE ” first, before moving on to the solution.
Iterative way :
1) Initialize start and end indexes as start = 0, end = n-1
2) In a loop, swap arr[start] with arr[end] and change start and end as follows :
start = start +1, end = end – 1
Another example to reverse a string:
Below is the implementation of the above approach :
- C++
- C
- Java
- Python
- C#
- PHP
// Iterative C++ program to reverse an array
#include <bits/stdc++.h>
using namespace std;
/* Function to reverse arr[] from start to end*/
void rvereseArray(int arr[], int start, int end)
{
while (start < end)
{
int temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
start++;
end--;
}
}
/* Utility function to print an array */
void printArray(int arr[], int size)
{
for (int i = 0; i < size; i++)
cout << arr[i] << " ";
cout << endl;
}
/* Driver function to test above functions */
int main()
{
int arr[] = {1, 2, 3, 4, 5, 6};
int n = sizeof(arr) / sizeof(arr[0]);
// To print original array
printArray(arr, n);
// Function calling
rvereseArray(arr, 0, n-1);
cout << "Reversed array is" << endl;
// To print the Reversed array
printArray(arr, n);
return 0;
}
Output :
1 2 3 4 5 6Reversed array is6 5 4 3 2 1
Time Complexity : O(n)
Recursive Way :
1) Initialize start and end indexes as start = 0, end = n-1
2) Swap arr[start] with arr[end]
3) Recursively call reverse for rest of the array.
Below is the implementation of the above approach :
- C++
- C
- Java
- Python
- C#
- PHP
// Recursive C++ program to reverse an array
#include <bits/stdc++.h>
using namespace std;
/* Function to reverse arr[] from start to end*/
void rvereseArray(int arr[], int start, int end)
{
if (start >= end)
return;
int temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
// Recursive Function calling
rvereseArray(arr, start + 1, end - 1);
}
/* Utility function to print an array */
void printArray(int arr[], int size)
{
for (int i = 0; i < size; i++)
cout << arr[i] << " ";
cout << endl;
}
/* Driver function to test above functions */
int main()
{
int arr[] = {1, 2, 3, 4, 5, 6};
// To print original array
printArray(arr, 6);
// Function calling
rvereseArray(arr, 0, 5);
cout << "Reversed array is" << endl;
// To print the Reversed array
printArray(arr, 6);
return 0;
}
Output :
1 2 3 4 5 6Reversed array is6 5 4 3 2 1
Time Complexity : O(n)
Another Approach: Using Python List slicing
- Python3
def reverseList(A):
print( A[::-1])
# Driver function to test above function
A = [1, 2, 3, 4, 5, 6]
print(A)
print("Reversed list is")
reverseList(A)
Output:
[1, 2, 3, 4, 5, 6]Reversed list is[6, 5, 4, 3, 2, 1]