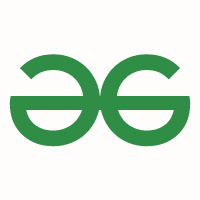
Variable Declaration and Scope in C - GeeksQuiz
C Variable Declaration and Scope
- Last Updated : 09 Aug, 2019
12
Question 1
Consider the following two C lines
int var1;
extern int var2;
Run on IDEWhich of the following statements is correct
A
Both statements only declare variables, don't define them.
B
First statement declares and defines var1, but second statement only declares var2
C
Both statements declare define variables var1 and var2
C Variable Declaration and Scope
Discuss it
Question 2
Predict the output
#include <stdio.h>
int var = 20;
int main()
{
int var = var;
printf("%d ", var);
return 0;
}
Run on IDE
A
Garbage Value
B
20
C
Compiler Error
C Variable Declaration and Scope
Discuss it
Question 3
#include <stdio.h>
extern int var;
int main()
{
var = 10;
printf("%d ", var);
return 0;
}
Run on IDE
A
Compiler Error: var is not defined
B
20
C
0
C Variable Declaration and Scope
Discuss it
Question 4
#include <stdio.h>
extern int var = 0;
int main()
{
var = 10;
printf("%d ", var);
return 0;
}
Run on IDE
A
10
B
Compiler Error: var is not defined
C
0
C Variable Declaration and Scope
Discuss it
Question 5
Output?
int main()
{
{
int var = 10;
}
{
printf("%d", var);
}
return 0;
}
Run on IDE
A
10
B
Compiler Error
C
Garbage Value
C Variable Declaration and Scope
Discuss it
Question 6
Output?
#include <stdio.h>
int main()
{
int x = 1, y = 2, z = 3;
printf(" x = %d, y = %d, z = %d n", x, y, z);
{
int x = 10;
float y = 20;
printf(" x = %d, y = %f, z = %d n", x, y, z);
{
int z = 100;
printf(" x = %d, y = %f, z = %d n", x, y, z);
}
}
return 0;
}
Run on IDE
A
x = 1, y = 2, z = 3x = 10, y = 20.000000, z = 3x = 1, y = 2, z = 100
B
Compiler Error
C
x = 1, y = 2, z = 3x = 10, y = 20.000000, z = 3x = 10, y = 20.000000, z = 100
D
x = 1, y = 2, z = 3x = 1, y = 2, z = 3x = 1, y = 2, z = 3
C Variable Declaration and Scope
Discuss it
Question 7
int main()
{
int x = 032;
printf("%d", x);
return 0;
}
Run on IDE
A
32
B
0
C
26
D
50
C Variable Declaration and Scope
Discuss it
Question 8
Consider the following C program, which variable has the longest scope?
int a;
int main()
{
int b;
// ..
// ..
}
int c;
Run on IDE
A
a
B
b
C
c
D
All have same scope
C Variable Declaration and Scope
Discuss it
Question 9
Consider the following variable declarations and definitions in C
i) int var_9 = 1;
ii) int 9_var = 2;
iii) int _ = 3;
Run on IDEChoose the correct statement w.r.t. above variables.
A
Both i) and iii) are valid.
B
Only i) is valid.
C
Both i) and ii) are valid.
D
All are valid.
C Variable Declaration and Scope C Quiz - 101
Discuss it
Question 10
Find out the correct statement for the following program.
#include "stdio.h"
int * gPtr;
int main()
{
int * lPtr = NULL;
if(gPtr == lPtr)
{
printf("Equal!");
}
else
{
printf("Not Equal");
}
return 0;
}
Run on IDE
A
It’ll always print Equal.
B
It’ll always print Not Equal.
C
Since gPtr isn’t initialized in the program, it’ll print sometimes Equal and at other times Not Equal.