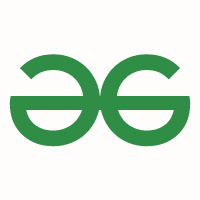
Use of bool in C - GeeksforGeeks
Use of bool in C
- Difficulty Level : Basic
- Last Updated : 14 Oct, 2020
Prerequisite: Bool Data Type in C++
The C99 standard for C language supports bool variables. Unlike C++, where no header file is needed to use bool, a header file “stdbool.h” must be included to use bool in C. If we save the below program as .c, it will not compile, but if we save it as .cpp, it will work fine.
- C
int main()
{
bool arr[2] = {true, false};
return 0;
}
If we include the header file “stdbool.h” in the above program, it will work fine as a C program.
- C
#include <stdbool.h>
int main()
{
bool arr[2] = { true, false };
return 0;
}
There is one more way to do it using enum function in C language. You can create a bool using enum. One enum will be created as bool, then put the elements of enum as True and False respectively. The false will be at the first position, so it will hold 0, and true will be at the second position, so it will get value 1.
Below is the implementation of the above idea:
- C
// C implementation of the above idea
#include <stdio.h>
// Declaration of enum
typedef enum { F, T } boolean;
int main()
{
boolean bool1, bool2;
bool1 = F;
if (bool1 == F) {
printf("bool1 is false\n");
}
else {
printf("bool1 is true\n");
}
bool2 = 2;
if (bool2 == F) {
printf("bool2 is false\n");
}
else {
printf("bool2 is true\n");
}
}
Output
bool1 is falsebool2 is true