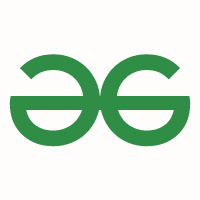
Unary operators in C/C++ - GeeksforGeeks
Unary operators in C/C++
- Difficulty Level : Basic
- Last Updated : 23 Nov, 2020
Unary operator: are operators that act upon a single operand to produce a new value.
Types of unary operators:
- unary minus(-)
- increment(++)
- decrement(- -)
- NOT(!)
- Addressof operator(&)
- sizeof()
- unary minus
The minus operator changes the sign of its argument. A positive number becomes negative, and a negative number becomes positive.int a = 10;int b = -a; // b = -10
unary minus is different from subtraction operator, as subtraction requires two operands.
- increment
It is used to increment the value of the variable by 1. The increment can be done in two ways:- prefix increment
In this method, the operator preceeds the operand (e.g., ++a). The value of operand will be altered before it is used.int a = 1;int b = ++a; // b = 2
- postfix increment
In this method, the operator follows the operand (e.g., a++). The value operand will be altered after it is used.int a = 1;int b = a++; // b = 1int c = a; // c = 2
- prefix increment
- decrement
It is used to decrement the value of the variable by 1. The decrement can be done in two ways:- prefix decrement
In this method, the operator preceeds the operand (e.g., – -a). The value of operand will be altered before it is used.int a = 1;int b = --a; // b = 0
- posfix decrement
In this method, the operator follows the operand (e.g., a- -). The value of operand will be altered after it is used.int a = 1;int b = a--; // b = 1int c = a; // c = 0
C++ program for combination of prefix and postfix operations:
// C++ program to demonstrate working of unary increment
// and decrement operators
#include <iostream>
using namespace std;
int main()
{
// Post increment
int a = 1;
cout << "a value: " << a << endl;
int b = a++;
cout << "b value after a++ : " << b << endl;
cout << "a value after a++ : " << a << endl;
// Pre increment
a = 1;
cout << "a value:" << a << endl;
b = ++a;
cout << "b value after ++a : " << b << endl;
cout << "a value after ++a : "<< a << endl;
// Post decrement
a = 5;
cout << "a value before decrement: " << a << endl;
b = a--;
cout << "b value after a-- : " << b << endl;
cout << "a value after a-- : " << a << endl;
// Pre decrement
a = 5;
cout << "a value: "<< a<<endl;
b = --a;
cout << "b value after --a : " << b << endl;
cout << "a value after --a : " << a << endl;
return 0;
}
Output:
a value: 1b value after a++ : 1a value after a++ : 2a value:1b value after ++a : 2a value after ++a : 2a value before decrement: 5b value after a-- : 5a value after a-- : 4a value: 5b value after --a : 4a value after --a : 4
The above program shows how the postfix and prefix works.
- prefix decrement
- NOT(!): It is used to reverse the logical state of its operand. If a condition is true, then Logical NOT operator will make it false.
If x is true, then !x is falseIf x is false, then !x is true
- Addressof operator(&): It gives an address of a variable. It is used to return the memory address of a variable. These addresses returned by the address-of operator are known as pointers because they “point” to the variable in memory.
& gives an address on variable nint a;int *ptr;ptr = &a; // address of a is copied to the location ptr.
- sizeof(): This operator returns the size of its operand, in bytes. The sizeof operator always precedes its operand.The operand is an expression, or it may be a cast.
#include <iostream>
using namespace std;
int main()
{
float n = 0;
cout << "size of n: " << sizeof(n);
return 1;
}
Output:
size of n: 4