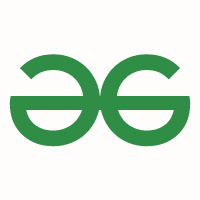
tolower() function in C - GeeksforGeeks
tolower() function in C
- Difficulty Level : Easy
- Last Updated : 01 Oct, 2018
The tolower() function is defined in the ctype.h header file. If the character passed is a uppercase alphabet then the tolower() function converts a uppercase alphabet to an lowercase alphabet.
Syntax:
int tolower(int ch);
Parameter: This method takes a mandatory parameter ch which is the character to be converted to lowercase.
Return Value: This function returns the lowercase character corresponding to the ch.
Below programs illustrate the tolower() function in C:
Example 1:-
// C program to demonstrate
// example of tolower() function.
#include <ctype.h>
#include <stdio.h>
int main()
{
// Character to be converted to lowercase
char ch = 'G';
// convert ch to lowercase using toLower()
printf("%c in lowercase is represented as = %c", ch, tolower(ch));
return 0;
}
Output:
G in lowercase is represented as = g
Example 2:-
// C program to demonstrate
// example of tolower() function.
#include <ctype.h>
#include <stdio.h>
int main()
{
int j = 0;
char str[] = "GEEKSFORGEEKS\n";
// Character to be converted to lowercase
char ch = 'G';
// convert ch to lowercase using toLower()
char ch;
while (str[j]) {
ch = str[j];
// convert ch to lowercase using toLower()
putchar(tolower(ch));
j++;
}
return 0;
}
Output:
geeksforgeeks