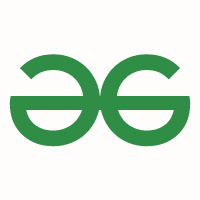
Rearrange array in alternating positive & negative items with O(1) extra space | Set 2 - GeeksforGeeks
Rearrange array in alternating positive & negative items with O(1) extra space | Set 2
- Difficulty Level : Medium
- Last Updated : 24 Aug, 2021
Given an array of positive and negative numbers, arrange them in an alternate fashion such that every positive number is followed by negative and vice-versa. Order of elements in output doesn’t matter. Extra positive or negative elements should be moved to end.
Examples:
Input :arr[] = {-2, 3, 4, -1}Output :arr[] = {-2, 3, -1, 4} OR {-1, 3, -2, 4} OR ..Input :arr[] = {-2, 3, 1}Output :arr[] = {-2, 3, 1} OR {-2, 1, 3}Input :arr[] = {-5, 3, 4, 5, -6, -2, 8, 9, -1, -4}Output :arr[] = {-5, 3, -2, 5, -6, 4, -4, 9, -1, 8} OR ..
Approach 1:
- First, sort the array in non-increasing order. Then we will count the number of positive and negative integers.
- Then swap the one negative and one positive number in the odd positions till we reach our condition.
- This will rearrange the array elements because we are sorting the array and accessing the element from left to right according to our need.
Below is the implementation of the above approach:
- C++
- Java
- Javascript
// Below is the implementation of the above approach
#include <bits/stdc++.h>
using namespace std;
// Function which works in the condition
// when number of negative numbers are
// lesser or equal than positive numbers
void fill1(int a[], int neg, int pos)
{
if (neg % 2 == 1)
{
for(int i = 1; i < neg; i += 2)
{
int c = a[i];
int d = a[i + neg];
int temp = c;
a[i] = d;
a[i + neg] = temp;
}
}
else
{
for(int i = 1; i <= neg; i += 2)
{
int c = a[i];
int d = a[i + neg - 1];
int temp = c;
a[i] = d;
a[i + neg - 1] = temp;
}
}
}
// Function which works in the condition
// when number of negative numbers are
// greater than positive numbers
void fill2(int a[], int neg, int pos)
{
if (pos % 2 == 1)
{
for(int i = 1; i < pos; i += 2)
{
int c = a[i];
int d = a[i + pos];
int temp = c;
a[i] = d;
a[i + pos] = temp;
}
}
else
{
for(int i = 1; i <= pos; i += 2)
{
int c = a[i];
int d = a[i + pos - 1];
int temp = c;
a[i] = d;
a[i + pos - 1] = temp;
}
}
}
// Reverse the array
void reverse(int a[], int n)
{
int i, k, t;
for(i = 0; i < n / 2; i++)
{
t = a[i];
a[i] = a[n - i - 1];
a[n - i - 1] = t;
}
}
// Print the array
void print(int a[], int n)
{
for(int i = 0; i < n; i++)
cout << a[i] << " ";
cout << endl;
}
// Driver code
int main()
{
int arr[] = { 2, 3, -4, -1, 6, -9 };
int n = sizeof(arr) / sizeof(arr[0]);
cout << "Given array is ";
print(arr, n);
int neg = 0, pos = 0;
for(int i = 0; i < n; i++)
{
if (arr[i] < 0)
neg++;
else
pos++;
}
// Sort the array
sort(arr, arr + n);
if (neg <= pos)
{
fill1(arr, neg, pos);
}
else
{
// Reverse the array in this condition
reverse(arr, n);
fill2(arr, neg, pos);
}
cout << "Rearranged array is ";
print(arr, n);
}
// This code is contributed by Potta Lokesh
Output
Given array is2 3 -4 -1 6 -9Rearranged array is-9 3 -1 2 -4 6
Time Complexity: O(N*logN)
Space Complexity: O(1)
Efficient Approach :
We have already discussed a O(n2) solution that maintains the order of appearance in the array here. If we are allowed to change order of appearance, we can solve this problem in O(n) time and O(1) space.
The idea is to process the array and shift all negative values to the end in O(n) time. After all negative values are shifted to the end, we can easily rearrange array in alternating positive & negative items. We basically swap next positive element at even position from next negative element in this step.
Following is the implementation of above idea.
- C++
- Java
- Python3
- C#
- PHP
- Javascript
// C++ program to rearrange
// array in alternating
// positive & negative items
// with O(1) extra space
#include <bits/stdc++.h>
using namespace std;
// Function to rearrange positive and negative
// integers in alternate fashion. The below
// solution doesn't maintain original order of
// elements
void rearrange(int arr[], int n)
{
int i = 0, j = n-1;
// shift all negative values to the end
while (i < j) {
while (i <= n - 1 and arr[i] > 0)
i += 1;
while (j >= 0 and arr[j] < 0)
j -= 1;
if (i < j )
swap(arr[i], arr[j]);
}
// i has index of leftmost
// negative element
if (i == 0 || i == n)
return;
// start with first positive
// element at index 0
// Rearrange array in alternating
// positive &
// negative items
int k = 0;
while (k < n && i < n ) {
// swap next positive
// element at even position
// from next negative element.
swap(arr[k], arr[i]);
i = i + 1;
k = k + 2;
}
}
// Utility function to print an array
void printArray(int arr[], int n)
{
for (int i = 0; i < n; i++)
cout << arr[i] << " ";
cout << endl;
}
// Driver code
int main()
{
int arr[] = { 2, 3, -4, -1, 6, -9 };
int n = sizeof(arr) / sizeof(arr[0]);
cout << "Given array is \n";
printArray(arr, n);
rearrange(arr, n);
cout << "Rearranged array is \n";
printArray(arr, n);
return 0;
}
Output:
Given array is2 3 -4 -1 6 -9Rearranged array is-1 3 -4 2 -9 6
Time Complexity : O(N)
Space Complexity : O(1)