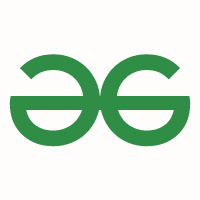
Program for Sum of the digits of a given number - GeeksforGeeks
Program for Sum of the digits of a given number
- Difficulty Level : Easy
- Last Updated : 03 Jun, 2021
Given a number, find sum of its digits.
Examples :
Input : n = 687Output : 21Input : n = 12Output : 3
Recommended: Please solve it on “PRACTICE ” first, before moving on to the solution.
General Algorithm for sum of digits in a given number:
- Get the number
- Declare a variable to store the sum and set it to 0
- Repeat the next two steps till the number is not 0
- Get the rightmost digit of the number with help of the remainder ‘%’ operator by dividing it by 10 and add it to sum.
- Divide the number by 10 with help of ‘/’ operator to remove the rightmost digit.
- Print or return the sum
Below are the solutions to get sum of the digits.
1. Iterative:
- C++
- C
- Java
- Python3
- C#
- PHP
- Javascript
// C program to compute sum of digits in
// number.
#include <iostream>
using namespace std;
/* Function to get sum of digits */
class gfg {
public:
int getSum(int n)
{
int sum = 0;
while (n != 0) {
sum = sum + n % 10;
n = n / 10;
}
return sum;
}
};
// Driver code
int main()
{
gfg g;
int n = 687;
cout << g.getSum(n);
return 0;
}
// This code is contributed by Soumik
Output
21
How to compute in a single line?
The below function has three lines instead of one line, but it calculates the sum in line. It can be made one-line function if we pass the pointer to sum.
- C++
- C
- Java
- Python3
- C#
- PHP
- Javascript
#include <iostream>
using namespace std;
/* Function to get sum of digits */
class gfg {
public:
int getSum(int n)
{
int sum;
/* Single line that calculates sum */
for (sum = 0; n > 0; sum += n % 10, n /= 10)
;
return sum;
}
};
// Driver code
int main()
{
gfg g;
int n = 687;
cout << g.getSum(n);
return 0;
}
// This code is contributed by Soumik
Output
21
2. Recursive
Thanks to Ayesha for providing the below recursive solution.
- C++
- C
- Java
- Python3
- C#
- PHP
- Javascript
// C++ program to compute
// sum of digits in number.
#include <iostream>
using namespace std;
class gfg {
public:
int sumDigits(int no)
{
return no == 0 ? 0 : no % 10 + sumDigits(no / 10);
}
};
// Driver code
int main(void)
{
gfg g;
cout << g.sumDigits(687);
return 0;
}
Output
21
3.Taking input as String
When the number of digits of that number exceeds 1019 , we can’t take that number as an integer since the range of long long int doesn’t satisfy the given number. So take input as a string, run a loop from start to the length of the string and increase the sum with that character(in this case it is numeric)
Below is the implementation of the above approach
- C++14
- Java
- Python3
- C#
- Javascript
// C++ implementation of the above approach
#include <iostream>
using namespace std;
int getSum(string str)
{
int sum = 0;
// Traversing through the string
for (int i = 0; i < str.length(); i++) {
// Since ascii value of
// numbers starts from 48
// so we subtract it from sum
sum = sum + str[i] - 48;
}
return sum;
}
// Driver Code
int main()
{
string st = "123456789123456789123422";
cout << getSum(st);
return 0;
}
Output
104
4. Using Tail Recursion
This problem can also be solved using Tail Recursion. Here is an approach to solving it.
1. Add another variable “Val” to the function and initialize it to ( val = 0 )
2. On every call to the function add the mod value (n%10) to the variable as “(n%10)+val” which is the last digit in n. Along with pass the variable n as n/10.
3. So on the First call it will have the last digit. As we are passing n/10 as n, It follows until n is reduced to a single digit.
4. n<10 is the base case so When n < 10, then add the n to the variable as it is the last digit and return the val which will have the sum of digits
- C++
- Java
- Python3
- C#
- Javascript
// C++ program for the above approach
#include <iostream>
using namespace std;
// Function to check sum
// of digit using tail recursion
int sum_of_digit(int n, int val)
{
if (n < 10)
{
val = val + n;
return val;
}
return sum_of_digit(n / 10, (n % 10) + val);
}
// Driver code
int main()
{
int num = 12345;
int result = sum_of_digit(num, 0);
cout << "Sum of digits is " << result;
return 0;
}
// This code is contributed by subhammahato348
Output
Sum of digits is 15