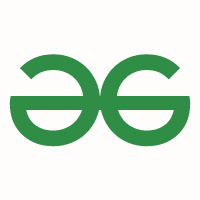
Predefined Identifier __func__ in C - GeeksforGeeks
Predefined Identifier __func__ in C
- Difficulty Level : Medium
- Last Updated : 11 May, 2020
Before we start discussing about __func__, let us write some code snippet and anticipate the output:
#include <stdio.h>
int main()
{
printf("%s",__func__);
return 0;
}
Will it compile error due to not defining variable __func__ ? Well, as you would have guessed so far, it won’t give any compile error and it’d print main!
C language standard (i.e. C99 and C11) defines a predefined identifier as follows in clause 6.4.2.2:
“The identifier __func__ shall be implicitly declared by the translator as if, immediately following the opening brace of each function definition, the declaration
static const char __func__[] = “function-name”;
appeared, where function-name is the name of the lexically-enclosing function.”
It means that C compiler implicitly adds __func__ in every function so that it can be used in that function to get the function name. To understand it better, let us write this code:
#include <stdio.h>
void foo(void)
{
printf("%s",__func__);
}
void bar(void)
{
printf("%s",__func__);
}
int main()
{
foo();
bar();
return 0;
}
And it’ll give output as foobar. A use case of this predefined identifier could be logging the output of a big program where a programmer can use __func__ to get the current function instead of mentioning the complete function name explicitly. Now what happens if we define one more variable of name __func__
#include <stdio.h>
int __func__ = 10;
int main()
{
printf("%d",__func__);
return 0;
}
Since C standard says compiler implicitly defines __func__ for each function as the function-name, we should not defined __func__ at the first place. You might get error but C standard says “undefined behaviour” if someone explicitly defines __func__ .
Just to finish the discussion on Predefined Identifier __func__, let us mention Predefined Macros as well (such as __FILE__ and __LINE__ etc.) Basically, C standard clause 6.10.8 mentions several predefined macros out of which __FILE__ and __LINE__ are of relevance here.
It’s worthwhile to see the output of the following code snippet:
#include <stdio.h>
int main()
{
printf("In file:%s, function:%s() and line:%d",__FILE__,__func__,__LINE__);
return 0;
}
Instead of explaining the output, we will leave this to you to guess and understand the role of __FILE__ and __LINE__!