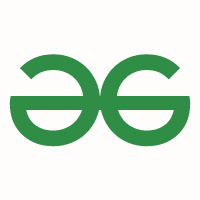
Pre-increment (or pre-decrement) in C++ - GeeksforGeeks
Pre-increment (or pre-decrement) in C++
- Difficulty Level : Medium
- Last Updated : 12 Jul, 2018
In C++, pre-increment (or pre-decrement) can be used as l-value, but post-increment (or post-decrement) can not be used as l-value.
For example, following program prints a = 20 (++a is used as l-value)
// CPP program to illustrate
// Pre-increment (or pre-decrement)
#include <cstdio>
int main()
{
int a = 10;
++a = 20; // works
printf("a = %d", a);
getchar();
return 0;
}
a = 20
The above program works whereas the following program fails in compilation with error “non-lvalue in assignment” (a++ is used as l-value)
// CPP program to illustrate
// Post-increment (or post-decrement)
#include <cstdio>
int main()
{
int a = 10;
a++ = 20; // error
printf("a = %d", a);
getchar();
return 0;
}
prog.cpp: In function 'int main()':prog.cpp:6:5: error: lvalue required as left operand of assignmenta++ = 20; // error ^
How ++a is different from a++ as lvalue?
It is because ++a returns an lvalue, which is basically a reference to the variable to which we can further assign — just like an ordinary variable. It could also be assigned to a reference as follows:
int &ref = ++a; // validint &ref = a++; // invalid
Whereas if you recall how a++ works, it doesn’t immediately increment the value it holds. For brevity, you can think of it as getting incremented in the next statement. So what basically happens is that a++ returns an rvalue, which is basically just a value like the value of an expression which is not stored. You can think of a++ = 20; as follows after being processed:
int a = 10;// On compilation, a++ is replaced by the value of a which is an rvalue:10 = 20; // Invalid// Value of a is incrementeda = a + 1;