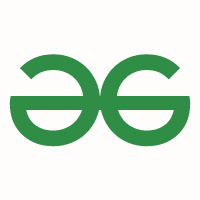
Power Function in C/C++ - GeeksforGeeks
Power Function in C/C++
- Difficulty Level : Easy
- Last Updated : 23 Aug, 2021
Given two numbers base and exponent, pow() function finds x raised to the power of y i.e. xy. Basically in C exponent value is calculated using the pow() function. pow() is function to get the power of a number, but we have to use #include<math.h> in c/c++ to use that pow() function. then two numbers are passed. Example – pow(4 , 2); Then we will get the result as 4^2, which is 16.
Example:
Input: 2.0, 5.0Output: 32Explanation: pow(2.0, 5.0) executes 2.0 raised tothe power 5.0, which equals 32Input: 5.0, 2.0Output: 25Explanation: pow(5.0, 2.0) executes 5.0 raised tothe power 2.0, which equals 25
Syntax:
double pow(double x, double y);
Parameters: The method takes two arguments:
- x : floating point base value
- y : floating point power value
Program:
C
// C program to illustrate
// power function
#include <math.h>
#include <stdio.h>
int main()
{
double x = 6.1, y = 4.8;
// Storing the answer in result.
double result = pow(x, y);
printf("%.2lf", result);
return 0;
}
C++
// CPP program to illustrate
// power function
#include <bits/stdc++.h>
using namespace std;
int main()
{
double x = 6.1, y = 4.8;
// Storing the answer in result.
double result = pow(x, y);
// printing the result upto 2
// decimal place
cout << fixed << setprecision(2) << result << endl;
return 0;
}
Output:
5882.79
Working of pow() function with integers
The pow() function takes ‘double’ as the arguments and returns a ‘double’ value. This function does not always work for integers. One such example is pow(5, 2). When assigned to an integer, it outputs 24 on some compilers and works fine for some other compilers. But pow(5, 2) without any assignment to an integer outputs 25.
- This is because 52 (i.e. 25) might be stored as 24.9999999 or 25.0000000001 because the return type is double. When assigned to int, 25.0000000001 becomes 25 but 24.9999999 will give output 24.
- To overcome this and output the accurate answer in integer format, we can add 0.5 to the result and typecast it to int e.g (int)(pow(5, 2)+0.5) will give the correct answer(25, in above example), irrespective of the compiler.
C
// C program to illustrate
// working with integers in
// power function
#include <math.h>
#include <stdio.h>
int main()
{
int a;
// Using typecasting for
// integer result
a = (int)(pow(5, 2) + 0.5);
printf("%d", a);
return 0;
}
C++
// CPP program to illustrate
// working with integers in
// power function
#include <bits/stdc++.h>
using namespace std;
int main()
{
int a;
// Using typecasting for
// integer result
a = (int)(pow(5, 2) + 0.5);
cout << a;
return 0;
}
Output:
25