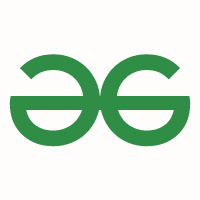
Move all zeroes to end of array | Set-2 (Using single traversal) - GeeksforGeeks
Move all zeroes to end of array | Set-2 (Using single traversal)
- Difficulty Level : Easy
- Last Updated : 03 May, 2021
Given an array of n numbers. The problem is to move all the 0’s to the end of the array while maintaining the order of the other elements. Only single traversal of the array is required.
Examples:
Input : arr[] = {1, 2, 0, 0, 0, 3, 6}Output : 1 2 3 6 0 0 0Input: arr[] = {0, 1, 9, 8, 4, 0, 0, 2, 7, 0, 6, 0, 9}Output: 1 9 8 4 2 7 6 9 0 0 0 0 0
Recommended: Please try your approach on {IDE} first, before moving on to the solution.
Algorithm:
moveZerosToEnd(arr, n)Initialize count = 0for i = 0 to n-1if (arr[i] != 0) thenswap(arr[count++], arr[i])
- CPP
- Java
- Python3
- C#
- Javascript
// C++ implementation to move all zeroes at
// the end of array
#include <iostream>
using namespace std;
// function to move all zeroes at
// the end of array
void moveZerosToEnd(int arr[], int n)
{
// Count of non-zero elements
int count = 0;
// Traverse the array. If arr[i] is non-zero, then
// swap the element at index 'count' with the
// element at index 'i'
for (int i = 0; i < n; i++)
if (arr[i] != 0)
swap(arr[count++], arr[i]);
}
// function to print the array elements
void printArray(int arr[], int n)
{
for (int i = 0; i < n; i++)
cout << arr[i] << " ";
}
// Driver program to test above
int main()
{
int arr[] = { 0, 1, 9, 8, 4, 0, 0, 2,
7, 0, 6, 0, 9 };
int n = sizeof(arr) / sizeof(arr[0]);
cout << "Original array: ";
printArray(arr, n);
moveZerosToEnd(arr, n);
cout << "\nModified array: ";
printArray(arr, n);
return 0;
}
Output:
Original array: 0 1 9 8 4 0 0 2 7 0 6 0 9Modified array: 1 9 8 4 2 7 6 9 0 0 0 0 0
Time Complexity: O(n).
Auxiliary Space: O(1).