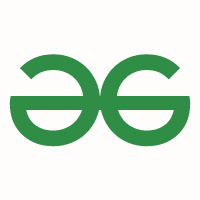
How to assign values to variables in Python and other languages - GeeksforGeeks
How to assign values to variables in Python and other languages
- Difficulty Level : Basic
- Last Updated : 07 Jul, 2021
This article discusses methods to assign values to variables.
Method 1: Direct Initialisation Method
- C++
- C
- Java
- Python3
- C#
- Javascript
// C++ code to demonstrate variable assignment
// upon condition using Direct Initialisation Method
#include <bits/stdc++.h>
using namespace std;
int main()
{
// initialising variables directly
int a = 5;
// printing value of a
cout << "The value of a is: " << a;
}
Output:
The value of a is: 5
Method 2: Using Conditional Operator (?:)
Below is the syntax in other popular languages.
- C++
- C
- Java
- Python3
- C#
- Javascript
// C++ code to demonstrate variable assignment
// upon condition using Conditional Operator
#include <bits/stdc++.h>
using namespace std;
int main()
{
// initialising variables using Conditional Operator
int a = 20 > 10 ? 1 : 0;
// printing value of a
cout << "The value of a is: " << a;
}
Output:
The value of a is: 1
One liner if-else instead of Conditional Operator (?:) in Python
- Python3
# Python 3 code to demonstrate variable assignment
# upon condition using One liner if-else
# initialising variable using Conditional Operator
# a = 20 > 10 ? 1 : 0 is not possible in Python
# Instead there is one liner if-else
a = 1 if 20 > 10 else 0
# printing value of a
print ("The value of a is: " + str(a))
Output:
The value of a is: 1