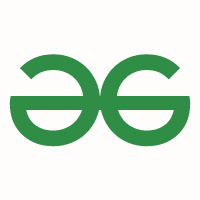
exit() vs _Exit() in C and C++ - GeeksforGeeks
exit() vs _Exit() in C and C++
- Difficulty Level : Medium
- Last Updated : 08 Jun, 2018
In C, exit() terminates the calling process without executing the rest code which is after the exit() function.
Example:-
// C program to illustrate exit() function.
#include <stdio.h>
#include <stdlib.h>
int main(void)
{
printf("START");
exit(0); // The program is terminated here
// This line is not printed
printf("End of program");
}
Output:
START
Now the question is that if we have exit() function then why C11 standard introduced _Exit()? Actually exit() function performs some cleaning before termination of the program like connection termination, buffer flushes etc. The _Exit() function in C/C++ gives normal termination of a program without performing any cleanup tasks. For example it does not execute functions registered with atexit.
Syntax:
// Here the exit_code represent the exit status// of the program which can be 0 or non-zero.// The _Exit() function returns nothing.void _Exit(int exit_code);
// C++ program to demonstrate use of _Exit()
#include <stdio.h>
#include <stdlib.h>
int main(void)
{
int exit_code = 10;
printf("Termination using _Exit");
_Exit(exit_code);
}
Output:
Showing difference through programs:
// A C++ program to show difference
// between exit() and _Exit()
#include<bits/stdc++.h>
using namespace std;
void fun(void)
{
cout << "Exiting";
}
int main()
{
atexit(fun);
exit(10);
}
Output
Exiting
If we replace exit with _Exit(), then nothing is printed.
// A C++ program to show difference
// between exit() and _Exit()
#include<bits/stdc++.h>
using namespace std;
void fun(void)
{
cout << "Exiting";
}
int main()
{
atexit(fun);
_Exit(10);
}