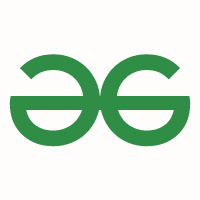
Debugging Python code using breakpoint() and pdb - GeeksforGeeks
Debugging Python code using breakpoint() and pdb
- Difficulty Level : Medium
- Last Updated : 23 Feb, 2019
While developing an application or exploring some features of a language, one might need to debug the code anytime. Therefore, having an idea of debugging the code is quite necessary. Let’s see some basics of debugging using built-in function breakpoint() and pdb module.
We know that debugger plays an important role when we want to find a bug in a particular line of code. Here, Python comes with the latest built-in function breakpoint which do the same thing as pdb.set_trace() in Python 3.6 and below versions.
Debugger finds the bug in the code line by line where we add the breakpoint, if a bug is found then program stops temporarily then you can remove the error and start to execute the code again.
Syntax:
1) breakpoint() # in Python 3.72) import pdb; pdb.set_trace() # in Python 3.6 and below
Method #1 : Using breakpoint() function
In this method, we simply introduce the breakpoint where you have doubt or somewhere you want to check for bugs or errors.
def debugger(a, b):
breakpoint()
result = a / b
return result
print(debugger(5, 0))
Output :
In order to run the debugger just type c and press enter.
Commands for debugging :
c -> continue executionq -> quit the debugger/executionn -> step to next line within the same functions -> step to next line in this function or a called function
Method #2 : Using pdb module
As the same suggests, PDB means Python debugger. To use the PDB in the program we have to use one of its method named set_trace(). Although this will result the same but this the another way to introduce the debugger in python version 3.6 and below.
def debugger(a, b):
import pdb; pdb.set_trace()
result = a / b
return result
print(debugger(5, 0))
Output :
In order to run the debugger just type c and press enter.
Example :
def debugger(a):
import pdb; pdb.set_trace()
result = [a[element] for element in range(0, len(a)+5)]
return result
print(debugger([1, 2, 3]))
Output :
Attention geek! Strengthen your foundations with the Python Programming Foundation Course and learn the basics.
To begin with, your interview preparations Enhance your Data Structures concepts with the Python DS Course. And to begin with your Machine Learning Journey, join the Machine Learning – Basic Level Course
Like
0
Check multiple conditions in if statement - Python
RECOMMENDED ARTICLES
Page :
Python | Basic Program Crash Debugging
Debugging decorators in Python
Performing Google Search using Python code
Python | Generate QR Code using pyqrcode module
Issues with using C code in Python | Set 1
Issues with using C code in Python | Set 2
Convert Python Code to a Software to Install on Windows Using Inno Setup Compiler
Application to get address details from zip code Using Python
Wi-Fi QR Code Generator Using Python
Get Bank details from IFSC Code Using Python
Create a GUI to search bank information with IFSC Code using Python
Get Zip Code with given location using GeoPy in Python
Get Indian Railways Station code Using Python
Generate QR Code using qrcode in Python
Auto Search StackOverflow for Errors in Code using Python
Convert HTML source code to JSON Object using Python
Python code formatting using Black
Python - Morse Code Translator GUI using Tkinter
Extract the HTML code of the given tag and its parent using BeautifulSoup
Packaging and Publishing Python code
Article Contributed By :