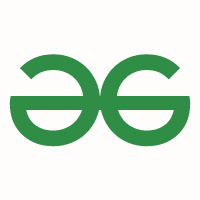
Data Types in C - GeeksQuiz
C Data Types
- Last Updated : 24 Sep, 2020
12
Question 1
Predict the output of following program. Assume that the numbers are stored in 2's complement form.
#include<stdio.h>
int main()
{
unsigned int x = -1;
int y = ~0;
if (x == y)
printf("same");
else
printf("not same");
return 0;
}
Run on IDE
A
same
B
not same
Question 2
Which of the following is not a valid declaration in C?
1. short int x;
Run on IDE
2. signed short x;
Run on IDE
3. short x;
Run on IDE
4. unsigned short x;
Run on IDE
A
3 and 4
B
2
C
1
D
All are valid
Question 3
Predict the output
#include <stdio.h>
int main()
{
float c = 5.0;
printf ("Temperature in Fahrenheit is %.2f", (9/5)*c + 32);
return 0;
}
Run on IDE
A
Temperature in Fahrenheit is 41.00
B
Temperature in Fahrenheit is 37.00
C
Temperature in Fahrenheit is 0.00
D
Compiler Error
Question 4
Predict the output of following C program
#include <stdio.h>
int main()
{
char a = 012;
printf("%d", a);
return 0;
}
Run on IDE
A
Compiler Error
B
12
C
10
D
Empty
Question 5
In C, sizes of an integer and a pointer must be same.
A
True
B
False
Question 6
Output?
int main()
{
void *vptr, v;
v = 0;
vptr = &v;
printf("%v", *vptr);
getchar();
return 0;
}
Run on IDE
A
0
B
Compiler Error
C
Garbage Value
Question 7
Assume that the size of char is 1 byte and negatives are stored in 2's complement form
#include<stdio.h>
int main()
{
char c = 125;
c = c+10;
printf("%d", c);
return 0;
}
Run on IDE
A
135
B
+INF
C
-121
D
-8
Question 8
#include <stdio.h>
int main()
{
if (sizeof(int) > -1)
printf("Yes");
else
printf("No");
return 0;
}
Run on IDE
A
Yes
B
No
C
Compiler Error
D
Runtime Error
Question 9
Suppose n and p are unsigned int variables in a C program. We wish to set p to nC3. If n is large, which of the following statements is most likely to set p correctly?
A
p = n * (n-1) * (n-2) / 6;
B
p = n * (n-1) / 2 * (n-2) / 3;
C
p = n * (n-1) / 3 * (n-2) / 2;
D
p = n * (n-1) * (n-2) / 6.0;
C Data Types GATE-CS-2014-(Set-2)
Discuss it
Question 10
Output of following program?
#include<stdio.h>
int main()
{
float x = 0.1;
if ( x == 0.1 )
printf("IF");
else if (x == 0.1f)
printf("ELSE IF");
else
printf("ELSE");
}
Run on IDE
A
ELSE IF
B
IF
C
ELSE