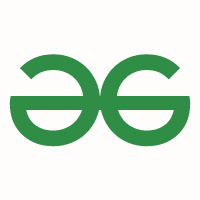
Dangling, Void , Null and Wild Pointers - GeeksforGeeks
Dangling, Void , Null and Wild Pointers
- Difficulty Level : Easy
- Last Updated : 28 Jun, 2021
Dangling pointer
A pointer pointing to a memory location that has been deleted (or freed) is called dangling pointer. There are three different ways where Pointer acts as dangling pointer
- De-allocation of memory
// Deallocating a memory pointed by ptr causes
// dangling pointer
#include <stdlib.h>
#include <stdio.h>
int main()
{
int *ptr = (int *)malloc(sizeof(int));
// After below free call, ptr becomes a
// dangling pointer
free(ptr);
// No more a dangling pointer
ptr = NULL;
}
- Function Call
// The pointer pointing to local variable becomes
// dangling when local variable is not static.
#include<stdio.h>
int *fun()
{
// x is local variable and goes out of
// scope after an execution of fun() is
// over.
int x = 5;
return &x;
}
// Driver Code
int main()
{
int *p = fun();
fflush(stdin);
// p points to something which is not
// valid anymore
printf("%d", *p);
return 0;
}
Output:
A garbage Address